NuxtZzle starterkit | Nuxt Better Auth - How to implement user verification
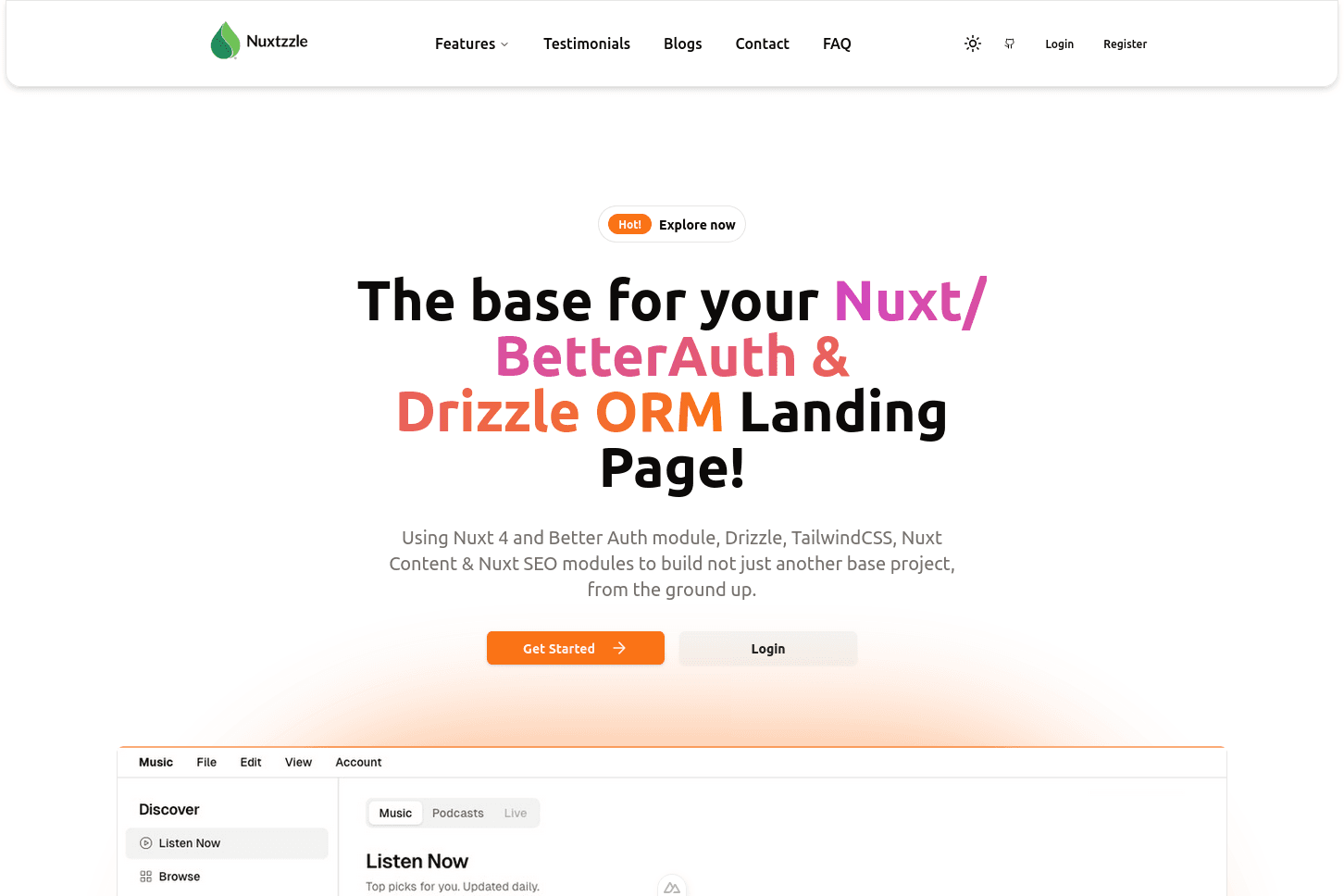
After user sing up, he can verify his email address by clicking on the link in his email.
- But we need to trigger that email verification.
In the lib/auth.ts
file, we need to add the following code:
emailVerification: {
async sendVerificationEmail(user, url) {
await sendUserVerificationEmail(user, url);
},
sendOnSignUp: true,
},
Where the sendUserVerificationEmail
function is defined in the /server/utils/email
file, in this function we will handle the following:
- Create the template for the email using mjml
- We pass the user information to the template generation plus the url that will containt eh verification link
- We send the email using the Mailgun API
Note Please make sure that you have the following environment variables set in your
.env
file:
MAILGUN_API_KEY
MAILGUN_DOMAIN
MAIL_FROM_EMAIL
Now that we implement the email verification we need to enforce the user to verify his email address by clicking on the link in his email.
We will need to update our lib/auth.ts
file.
emailAndPassword: {
requireEmailVerification: true
}
Overview of the user registration flow:
- User sign up
- We send a verification email
- User clicks on the link in the email to verify his email address
- User try to login
If the user try to login with out verifying his email address, the login will fail.
We will handle that in the /pages/login.vue
file. By showing a message to the user that he need to verify his email address.
When the user submits the form to login we just need to handle the onError
from the signIn.email
function.
await signIn.email({
//Rest of the code here...
fetchOptions: {
onError: (context) => {
toast({
title: "Please try again",
description: context?.error?.message || "Please check your email and password",
variant: "destructive",
});
},
},
});
This will display a toast message to the user that he need to verify his email address or the error message generated by the Better Auth
.
Note
When the user try to register with a existing email address
A more generic error message will be better. that way we don't show if the email is in out system, but it will be better to send a verification email base on the existing email address. and let the user know that a new registration attempt has been made.
The email for the verification is the following: